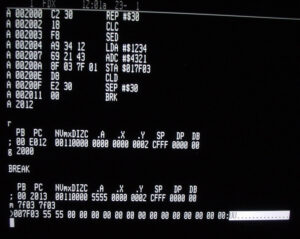
Meet picoVM. A fully featured, minimal footprint, VM for embedded devices.
Written in pure C for maximum efficiency and portability. Essentially just a single header file and one source file that you can include in your project.
The machine supports 8,16 and 32bit integer operations along with floating point numbers. You get this at a 1000 bytes footprint, and by removing unnecessary features you can get down to 700 bytes.
You can get the source code at my GitHub page here. Along the source code you can find examples that make use of the VM, along with a -crude- assembler.
Here is what a hello world program looks like in picoVM:
; ; Hello world example in picoVM assembly ; loop: LOAD16 message ; load the message address LOAD16 [idx] ; load the index LOAD ; using the loaded address and index load the char JEQ finish ; if zero the string terminated CALLUSER ; print the character POP ; pop the character LOAD [idx] ; Increase the index LOAD 1 ; ADD ; STORE [idx] ; JMP loop ; repeat finish: HLT idx: DB 0x0000 message: DB "Hello World!" DB 0x00
Yes, I know, I just put assembly code in front of your eyes. I am not sorry! There are more advanced examples in the source code, if you are interested.
Now, lets see who will create an LLVM backend for this… Have fun!